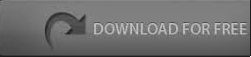

- #PYTHON IZIP PYTHON 3 INSTALL#
- #PYTHON IZIP PYTHON 3 CODE#
- #PYTHON IZIP PYTHON 3 SERIES#
- #PYTHON IZIP PYTHON 3 ZIP#
If you want to merge lists into a list of tuples or zip two lists, you can use the zip() method. You can get a list from it by calling list(zip(a, b)). In python 3.0, the zip method returns the zip object. We can also convert the output to the tuple.
#PYTHON IZIP PYTHON 3 CODE#
Write the following code inside the app.py file. Imagine drawing a zipper horizontally from left to right.

a: a1 a2 a3 a4 a5 a6 a7.Īnd “zip” them into one list whose entries are 3-tuples (ai, bi, ci). The zip() function takes the list like the following. If you’re going to use a Python zip() function with the unordered iterables like sets, this is something to consider. Unfortunately, this means that the tuples returned by the zip() will have items that are paired up randomly. In this example, setA and setB are set objects which don’t keep their items in any particular order. Now, you zip the list and convert the zipped object back to the list, but the values will lose their orders this time. For example, if there are three items in two sets. The result might be weird if you’re working with sequences like sets. So let’s define two strings, pass those in the zip() function, and see the output. If you’re working with sequences like the strings, your iterables will be evaluated from left to right. You call a Python zip method with three iterables(list of strings, integers, and booleans), so the resulting tuples have three elements. You see that the length of the resulting tuples will always equal the number of iterables you pass as arguments.
#PYTHON IZIP PYTHON 3 SERIES#
Python zip() function can take just one argument and returns an iterator that yields a series of 1-item tuples. However, since zipped holds the empty iterator, there’s nothing to pull out, so Python raises the StopIteration exception. Python tries to retrieve the next item when you call the next() on zipped. In this case, you’ll get a StopIteration exception. You could also force the empty iterator to yield an element directly. Likewise, if you consume the iterator with a list(), you’ll see an empty list. You call zip() with no arguments, so your zipped variable holds an empty iterator. Thus, to retrieve a final list object, you need to use the list() to consume the iterator. Notice how the Python zip() function returns the iterator. The x values are taken from the numbers, and the y values are taken from letters. Here, you use the zip(numbers, letters) to create the iterator that produces tuples of the form (x, y). For example, if you use the zip() with n arguments, that function will return the iterator that generates tuples of length n. The type() is a built-in function that returns the Python object type. To check the zip object in Python, use the type() function. It’s because the iterator stops when the shortest iterable is exhausted. Then, a returned iterator has three tuples. If multiple iterables are passed, i th tuple contains i th Suppose, and two iterables are given, one iterable containing 3 and the other containing five elements.Meaning, the number of items in each tuple is 1. If a single iterable is passed to the zip function, zip() returns the iterator of 1-tuples.If no parameters are passed on the zip function, zip() returns the empty iterator.

The zip() function returns the iterator of tuples based on an iterable object.

Iterables – can be built-in iterables (like a list, string, dict) or user-defined iterables (an object with an _iter_ method). The syntax of the zip() function in Python is the following.
#PYTHON IZIP PYTHON 3 INSTALL#
For Python3 users, pip install ipython will be just fine. If you are not using IPython, install it: “ pip3 install ipython” as I use Python 3. If you are using IPython, then type zip? And check what zip() is about. If the zip python function gets no iterable items, it returns the empty iterator.
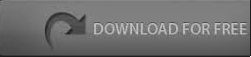